背景描述
最近开发中遇到需求,需要实现图片的格子分布效果,如下图所示

其中要求:
- 图片与图片的间隔,图片与屏幕的左边距,以及图片与屏幕的右边距,都为固定大小,比如
10dp
。
- 图片宽高相等。
代码实现
主要代码如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val images = listOf(...) val imageAdapter = ImageAdapter(images) rv_images.apply { layoutManager = GridLayoutManager(context, 4, RecyclerView.VERTICAL, false) addItemDecoration(EvenItemDecoration(dp2px(context, 10), 4)) adapter = imageAdapter } }
class ImageAdapter(private val images: List<Int> = listOf()) : RecyclerView.Adapter<ImageAdapter.ViewHolder>() { override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder { val view = LayoutInflater.from(parent.context).inflate(R.layout.item_image, parent, false) val ivSize = (getScreenContentWidth(parent.context) - dp2px(parent.context, 10) * 5) / 4 view.iv_image.layoutParams.height = ivSize return ViewHolder(view) }
override fun onBindViewHolder(holder: ViewHolder, position: Int) { holder.view.iv_image.setImageResource(images[position]) } ... }
class EvenItemDecoration(private val space: Int, private val column: Int) : RecyclerView.ItemDecoration() { override fun getItemOffsets(outRect: Rect, view: View, parent: RecyclerView, state: RecyclerView.State) { val position = parent.getChildAdapterPosition(view) val colPadding = space * (column + 1) / column val colIndex = position % column outRect.left = space * (colIndex + 1) - colPadding * colIndex outRect.right = colPadding * (colIndex + 1) - space * (colIndex + 1) if (position >= column) { outRect.top = space } } }}
|
布局item_image.xml
如下:
1 2 3 4 5 6 7 8 9 10
| <?xml version="1.0" encoding="utf-8"?> <ImageView android:id="@+id/iv_image" xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:scaleType="fitXY" tools:src="@mipmap/ic_launcher" android:background="#ffccff"/>
|
其中需要注意是
- 图片的宽度在布局和代码中不能写死,需要自适应。
- 在代码(Adapter)中根据屏幕宽度来计算图片高度。
- 在ItemDecoration中计算每列的左右间隙。
分析-GridLayoutManger分配Item空间的机制
GridLayoutManger在绘制子View的时候,会先为它们分配固定的空间。
比如,我们这里是4列,则每列分配父布局宽度(这里是屏幕宽度) / 4的宽度大小的空间,每列占据的宽度相等。
- 每列由图片和空隙组成。空隙包括左空隙和右空隙,分布在图片的左右。
- 每列中的图片会局限在列分配的空间内,不会超出这个空间。如果图片宽度小于列宽,则剩余的宽度会分配给空隙;如果图片的宽度大于列宽,则不会有空隙,且图片超出列的部分会被遮盖。
- 如果使用ItemDecoration设置间隙,则间隙不会被压缩,如果图片过宽,则会占用当前图片的空间,图片会被压缩。
示例一-设置固定大宽度
在Adapter代码中设置图片的宽度并在去掉ItemDecoration
1 2 3 4 5 6 7 8 9 10 11
| ... val ivSize = (getScreenContentWidth(parent.context) - dp2px(parent.context, 10) * 5) / 4 view.iv_image.layoutParams.width = 1000 view.iv_image.layoutParams.height = ivSize ...
...
...
|
结果如下:

通过Studio自带Layout Inspector
可以查看每个图片的左右位置,第一行图片的mLeft
分为0、360、720、1080,对应的mRight
是mLeft
+ 1000。
以上示例说明
- 每个图片占据的父布局的宽度是一样的,为父布局宽度除以列数,这里是1440 / 4 = 360。
- 每个图片超出所占据空间宽度后的部分会被后面的图片或屏幕遮盖。
示例二-设置较大的左右间隔
修改EvenItemDecoration代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| rv_items.apply { layoutManager = GridLayoutManager(context, 4, RecyclerView.VERTICAL, false) addItemDecoration(EvenItemDecoration(dp2px(context, 20), 4)) adapter = imageAdapter }
class EvenItemDecoration(private val space: Int, private val column: Int) : RecyclerView.ItemDecoration() { override fun getItemOffsets(outRect: Rect, view: View, parent: RecyclerView, state: RecyclerView.State) { outRect.left = space outRect.right = space } }
|
运行结果如下:
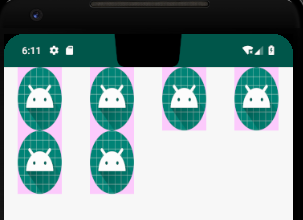
通过Layout Inspector
查看每个图片的左右位置,每个图片的大小为220,左右间隙都为70(px/dp=3.5),说明设置的间隙会占用图片之前分配的空间。
最后,根据以上机制,不难理解EvenItemDecoration
中的代码。
Github源代码地址:https://github.com/zhangliangnbu/gridlayout-demo